golang类型转换(指定精度/四舍五入)
  热度 °
Go
的数据类型很多都需要显示转换才能使用,比如string
转float64
指定精度等。转换中常用到的第三方包为strconv
和math
包。
整型转字符串
1 | strconv.Itoa(i) //方法1 |
字符串转整型
1 | strconv.Atoi(s) //方法1 |
bytes转float64
1 | func bytesToFloat64(bytes []byte) float64 { |
float64转bytes
1 | func float64ToBytes(input float64) []byte { |
float64转string
1 | func FloatToStr(num float64, floatPartLen int) string { |
string转float64
string
转float64
这里有两种方法,都支持指定精度。 注意:所有数字要在表现层显示最好转换为字符串传送给表现层,如果用于后端计算则转换为数字即可。比如:数字2.10 如果用保持5位数字精度显示: 那么 数字2.10 显示为:2.1, 而将2.10转换为字符串同时保持5位精度,则显示为: 2.10000。但是它们都是转换为了5位精度的,只是显示的时候,数字2.10000 直接显示为2.1了, 所以要显示精度则转换为字符串,要用于计算则转换为数字。
方法1: 只支持指定精度1
2
3
4
5
6
7func strToFloat64(str string, len int) float64 {
lenstr := "%." + strconv.Itoa(len) + "f"
value,_ := strconv.ParseFloat(str,64)
nstr := fmt.Sprintf(lenstr,value)
val,_ := strconv.ParseFloat(nstr,64)
return val
}
方法2:支持指定精度,支持是否四舍五入1
2
3
4
5
6
7
8
9
10
11
12func strToFloat64Round(str string, prec int, round bool) float64 {
f,_ := strconv.ParseFloat(str,64)
return Precision(f,prec,round)
}
func Precision(f float64, prec int, round bool) float64 {
pow10_n := math.Pow10(prec)
if round {
return math.Trunc(f + 0.5/pow10_n)*pow10_n) / pow10_n
}
return math.Trunc((f)*pow10_n) / pow10_n
}
具体请参考[示例代码]
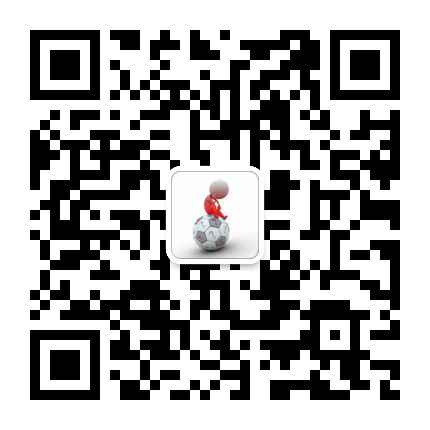
作者署名:朴实的一线攻城狮
本文标题:golang类型转换(指定精度/四舍五入)
本文出处:http://researchlab.github.io/2016/03/24/go-type-convert/
版权声明:本文由Lee Hong创作和发表,采用署名(BY)-非商业性使用(NC)-相同方式共享(SA)国际许可协议进行许可,转载请注明作者及出处, 否则保留追究法律责任的权利。